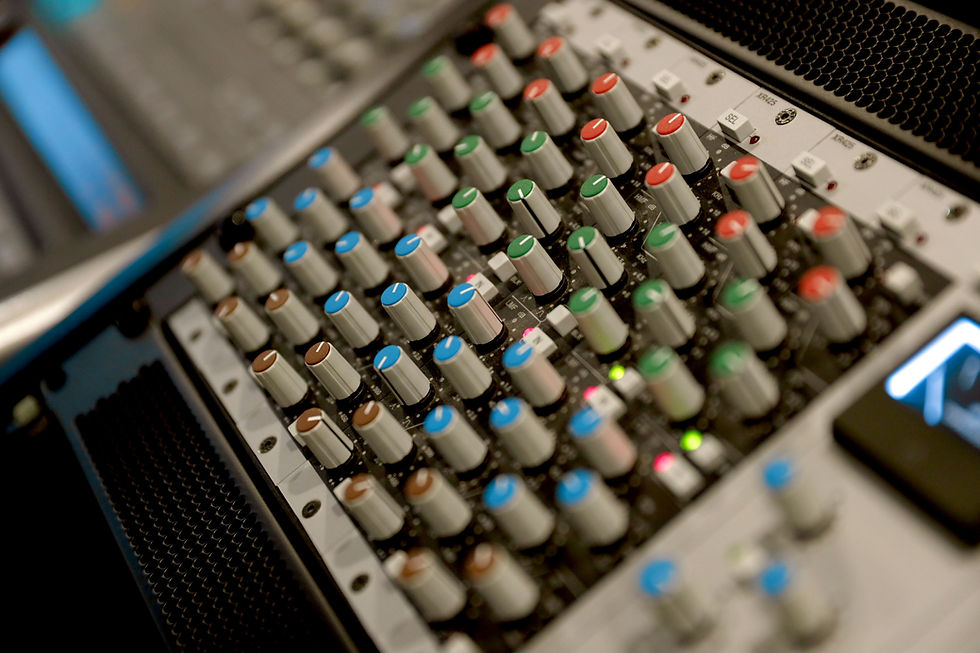
Let's talk about the logic of the frequency of characters.
Before thinking about logic, just how you will do it using pen and paper.
Let's say our word in "tommy" so, you will definitely write 't' on your paper and put value 1 in front of and so on.
Final result will look like this:
t -> 1
o -> 1
m -> 2
y -> 1
So, this is how you can solve this on paper.
Let's talk about the constraints of this program:
word will contain only alphabets(a-z)
All words will be lower case
The logic of Frequency Program:
So, First we have to store each and every number and count its frequecy as it is decided that there can be alphabets of 26 types.
Now, we have to create 26 counter variable and assign every variable for specified character.
But, 26 variables are not easy to handle so, instead of using 26 variable we can use array of size 26 and every index of that array will represent specified character.
for eg: int[] freq = new int[26]
So, we now problem is how to store our character counting the array. For that we have to extract characters from given string one by one.
For extracting characters from string we can use charAt, toCharArray methods in java.
Now, next hurdle is how to increase our count and how we can check which index is for which character.
For this part let's make a reference that index 0 will point to count of a, index 1 will point to count b, and so on upto z.
Now, if get 'p' as character how we wll get index for 'p'. So, as we defined as index 0 will point to 'a' so we have to count from character 'a' or we can find it by substracting 'a' from p will give index of our charcter in array frq.
This is how our code will work.
Check out this Code below:
import java.util.ArrayList;
import java.util.List;
public class CharacterFrequecy {
public static void main(String[] args) {
solve("vivek");
}
public static void solve(String name){
int[] frq = new int[26];
for(int i=0;i<name.length();i++){
frq[name.charAt(i)-'a']++;
}
for(int i=0;i<26;i++){
if(frq[i]!=0){
System.out.println((char)(i+'a')+" "+frq[i]);//line 1
}
}
}
}
//Output: i 1
k 1
v 2
Note: In line 1 we are type casting of int to char.
Comentários