Here, we will focusing on learning basics of coding in Java language
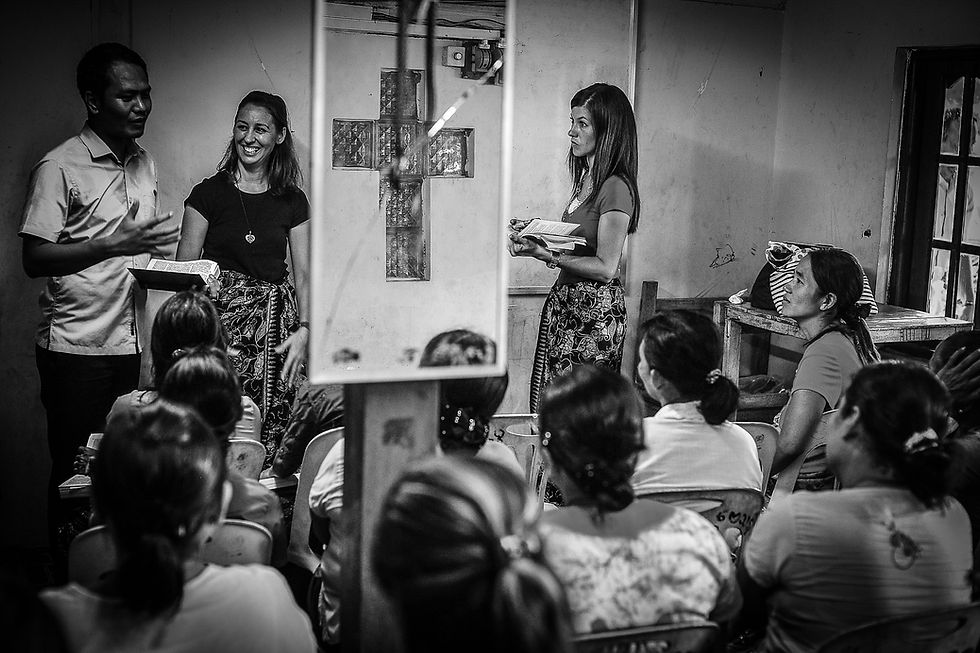
Before getting started, open your IDE where, you will practice same code and try to learn which particular part of code affect the whole program.
Start with Class name in Java
When we are dealing with coding in Java, main problem occurs with the class name of program.
In almost every coding platform your class main must be public and name should be "Main".
public class Main {
public static void main(String[] args) {
//your code
}
}
Getting Inputs
Programming approach starts from inputs
Generally, there are number of test-cases that you have to pass in your code.
Before getting values focus on type of input. Let's say if your inputs are of type string you follow same procedure as mentioned below will create problem for you. In the below scenario it string will we getting empty space.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// number of testcases
int testCase = sc.nextInt();
//loop for iterating over each testcase
while(testCase-- >0){
String str = sc.nextLine();
}
}
}
To remove this problem you can use the below code instead of any other approach.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// number of testcases
int testCase = Integer.parseInt(sc.nextLine());
//loop for iterating over each testcase
while(testCase-- >0){
//reduce one everytime and check for greaor than 0
String str = sc.nextLine();
// other inputs are also put inside this loop
}
}
}
This is all about taking inputs for our code.
Comments