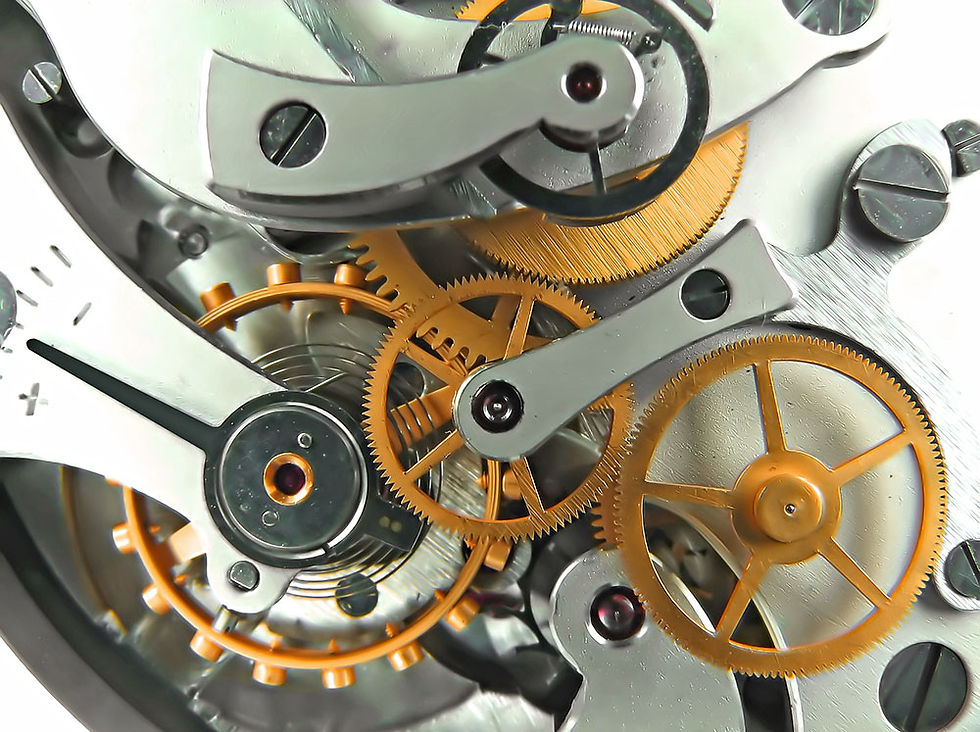
1. Conditional Statements
IF Statement
Inside IF statement you have to write valid boolean value(0, 1 works only in C Programming Language) value or statements returning boolean value.
int a =10, b=20;
boolean flag = false;
if(a==b) // true
if(a>=b) // false
if(flag)// false
2. Loops
For Loop
For loop comprised of three things initialization, condition(must be boolean type), and iteration. In all them condition to mandatory to apply. Initialization, condition and iteration can be blank inside for loop but, should be happen at some other place.
Used when the count of cycle is known.
public class Main{
public static void main (String[] args) {
int i=0;
for(;;){
i++;
if(i>5)break;
System.out.print(i);
}
}
}
//Output: 12345
While Loop
This loop requires only condition(must be boolean type) iteration and and initialization is upto user requirement. It uses basically uses when the count of cycle is not defined or known.
public class Main{
public static void main (String[] args) {
int i=5;
while(i-->0){
System.out.print(i);
}
}
}
//output:43210
Do while
Same like while but, first iteration happens before checking condition. Basically used where we have to perform at least one time mandatorily.
public class Main{
public static void main (String[] args) {
int i=5;
do{
System.out.print(i);
}while(i>5);
}
}
//output: 5
Comments