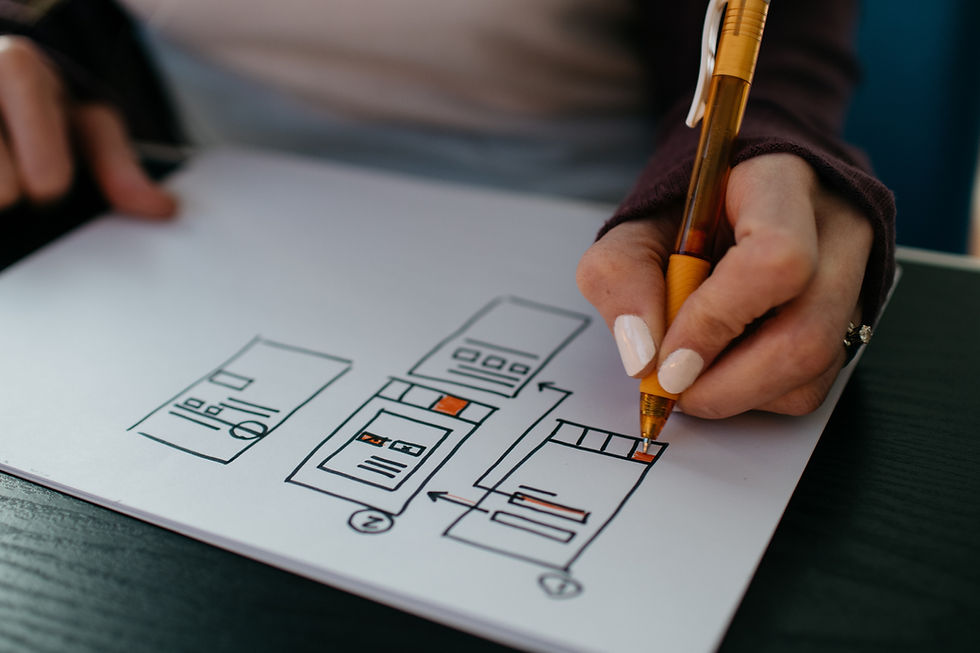
Before learning stream you should have knowledge of some functional interfaces.
let's discuss about these functional interfaces in this post.
Consumer Interface
It has one abstract method accept and one default method, and one default method.
void accept(T t);
default Consumer<T> andThen(Consumer<? super T> after) {
Objects.requireNonNull(after);
return (T t) -> { accept(t); after.accept(t); };
}
This method takes input can do manipulation but cannot return values. We can only print values using this method.
Note: forEach method of Java Streams using this method internally.
2. Predicate Interface
It has abstract method with name test, 3 default and 1 static method.
boolean test(T t);
default Predicate<T> and(Predicate<? super T> other) {
Objects.requireNonNull(other);
return (t) -> test(t) && other.test(t);
}
default Predicate<T> negate() {
return (t) -> !test(t);
}
default Predicate<T> or(Predicate<? super T> other) {
Objects.requireNonNull(other);
return (t) -> test(t) || other.test(t);
}
static <T> Predicate<T> isEqual(Object targetRef) {
return (null == targetRef)
? Objects::isNull
: object -> targetRef.equals(object);
}
Abstract method is of type boolean and can accepts parameter So, this method is basically uses for checking the condition and can get true or false based on the condition.
Note: Java Stream filter method uses this method internally.
3. Supplier Interface
This Interfaces contains one abstract method get.
T get();
This method do not accepts parameter and having return type.
Note: Java Stream uses this method in case where we want to get defined output.
Comments