Lambda in Java
- Vivek Bhardwaj
- Oct 2, 2021
- 1 min read
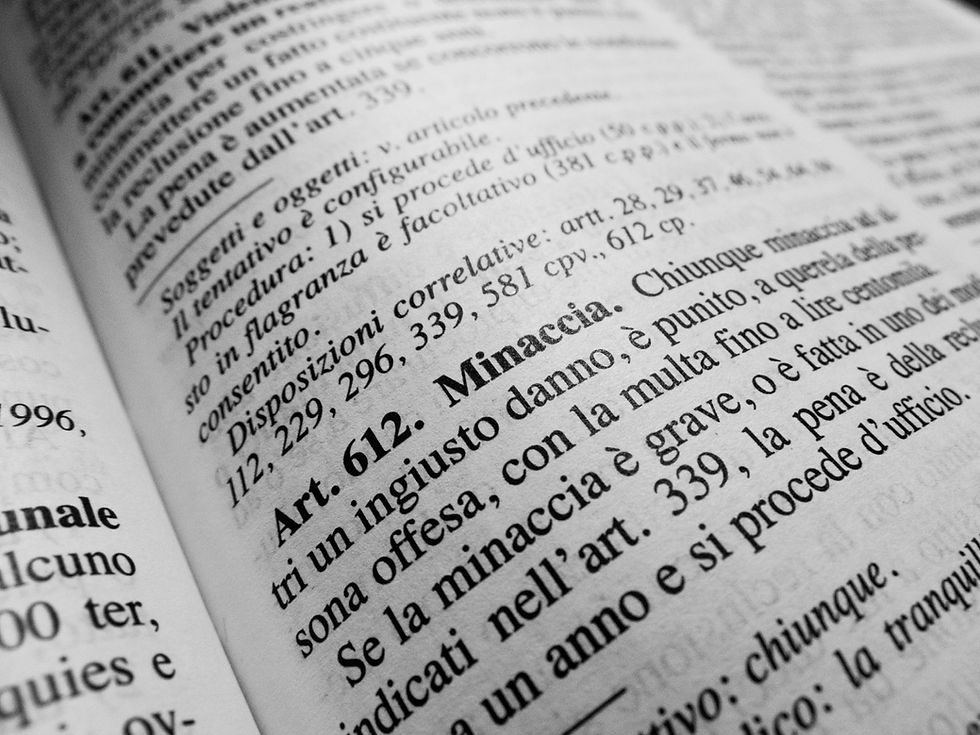
Let's talk about Java 8 feature Lambda expression. It is only valid for Functional Interface, an Interface having only one abstract method and can be any static and default methods. It is very useful while coding before Lambda we had to create class and implement abstract method available in the interface as given below:
interface Functional{
public int sum(int a, int b);
}
class Implement implements Functional{
@Override
public int sum(int a, int b){
return a+b;
}
}
public class Main
{
public static void main(String[] args) {
Functional fun = new Implement();
System.out.println(fun.sum(2,3));
}
}
Before coming to lambda let's talk about its representation.
We also called lambda expression as Anonymous method, having no name. So, as its name suggest we don't provide its name. Simple structure of lambda is given below:
(parameter list) -> {body}
let's implement the same method using lambda expression.
interface Functional{
public int sum(int a, int b);
}
public class Main
{
public static void main(String[] args) {
Functional fun = (a, b) -> a+b;
System.out.println(fun.sum(2,3));
}
}
So, above is the implementation with lambda expression. As you can see by using lambda expression we can reduce code.
Comentarios